In this post, I show different ways to generate a list and array containing numbers (ints, and floats, positive and negative, mixed) in python…using random, list, and numpy arrays.
Why do we need both? NumPy arrays are very efficient for numerical operations and require elements to be of the same data type, whereas lists are mutable and can contain elements of different types. For general-purpose storage we use lists and use NumPy arrays for intensive numerical computations. The numpy arrays and lists are different types of objects, so work with their values we have to convert them into the other type. For example, use numpy.tolist() to convert from a numpy array to a python list.
Here are some example codes…
Using direct assignment with simple list:
my_list = [1, 5, 3, 8.5, 2.7] # Mixed types
Using range(), generating lists within a scope:
my_integer_list = list(range(5)) # Integers from 0 to 4
my_range_list = list(range(2, 11, 2)) # Even integers from 2 to 10
Using list comprehension:
my_squares = [x**2 for x in range(10)] # Squares of numbers from 0 to 9
Using a loop, append(): ensuring all numbers have equal chance of being picked randomly using uniform()
import random
my_float_list = []
for i in range(5):
my_float_list.append(random.uniform(0, 10))
Using array.array():
import array
my_int_array = array.array('i', [5, 7, 2, 9]) # Integer array
my_float_array = array.array('d', [3.14, 1.59, 2.71]) # Float array
Using NumPy:
import numpy as np
#Direct assignment…my_array = np.array([4, 6, 2, 8.2, 1.5])
#Using arange()…my_integer_array = np.arange(10) # Integers from 0 to 9
#Using linspace()…my_float_array = np.linspace(0, 1, 5) # 5 evenly spaced floats from 0 to 1
#Using random functions…my_random_ints = np.random.randint(1, 10, size=5) # 5 random integers from 1 to 9
my_random_floats = np.random.random(5) # 5 random floats between 0 and 1
#Generate random integers within a range with specified number of items.sequence1 = np.random.randint(100, size=10) # between 1 and 99; containing 10 elements
#Generate a mix of numbers that can be positive, negative, integer, and float within a range and with specified number of items…
sequence2 = np.random.uniform(-99, 99, size=10) # Generate 10 random numbers between -99 and 99 (inclusive)
Numpy array have many decimal places for accuracy. Sometimes, we don’t need that precision and/or want to display only 2 decimal places. In such cases, use numpy.round(array, n) as shown below:
rounded_sequence2 = np.round(sequence2, 2) # rounded_sequence2 contains values upto 2 decimal places
#Convert a numpy array to a list…
list2 = rounded_sequence2.tolist() # list2 becomes a python list containing elements from numpy array with 2 decimal places
So, I showed how to convert a numpy array to a list, but what the other way?
There are two main ways to convert a list to a NumPy array: Using np.array(), and using np.asarray().
Use np.array() when you want to ensure a new NumPy array is created with a specific data type.
Use np.asarray() if you’re concerned about performance and the list data might already be contiguous in memory…it’s similar to np.array(), but it tries to avoid creating a new copy of the data if possible. This can be useful if the list is already stored in a contiguous memory block.
np.array() Example:import numpy as np
my_list = [1, 2.5, 3, 4.7]
# my_array is the new converted NumPy array from python list my_list
my_array = np.array(my_list)
np.asarray() Example:my_list = [1, 2.5, 3, 4.7]
my_array = np.asarray(my_list)
print(my_array is my_list) # Check: This will likely print False
▛Interested in creating programmable, cool electronic gadgets? Give my newest book on Arduino a try: Hello Arduino! 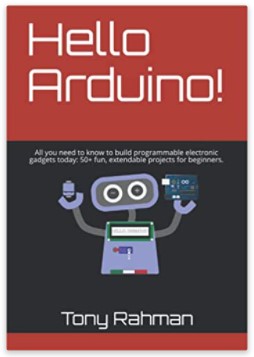
▟