In this post, I’ll touch on how appointments, events are commonly shared in the digital world and how to create, manipulate, and open such objects using simple code. But first, and introduction to iCalendar.
iCalendar is a file format used for exchanging calendar and scheduling information between computers. It’s the most universal and portable format used by virtually all calendar applications, including Apple Calendar, Microsoft Outlook, Google Calendar, and more.
Underneath, an iCalendar file is a plain text file that adheres to a specific syntax much like a HTML file except it is defined by the iCalendar specification. Typically, it has the .ics
file extension. Inside it, there’s information about an appointment or event including the start and end dates, times, location, description and recurrence. Because it is a standard format, a person can create an event in MacOS or a mobile application and send it to a Linux user, who can share it with a Windows user, and all that information remains intact and everyone can save the associated event information on their calendars (for work or personal purposes). It’s a really simple format and Python makes it very convenient to create such files, extract and parse the information from them, open them and more from within the code without even having to use any other client application. And that’s what I’ll demonstrate in this post.
HOW TO EXTRACT INFORMATION FROM AN EVENT (ICS) FILE USING CODE
Suppose you scheduled a massage appointment and you get an email confirmation of the time and location; you’ll most likely also get a link or attachment or some option to save that appointment to a file. That file, once you save it is actually the ICS file. Once you download and open it, your calendar application (whether a mobile Outlook or Office Outlook or anything else) will open where you can save it…meaning, it’ll go into your calendar application. But you can also parse the information without having to actually open it using another application using Python code as long as the ICS file is saved.
Let’s start with an example. An example ICS file content is shown below:
BEGIN:VCALENDAR
VERSION:2.0
PRODID:https://flyingsalmon.net
BEGIN:VEVENT
DTSTAMP:60 min Massage Session
DTSTART:20230209T130000
DTEND:20230209T140000
LOCATION: 15111 Main Street,Home City, WA 99999
DESCRIPTION:60 min massage session with Jen at 1:00 pm (555-555-5555).\nPlease plan to arrive 10-15 minutes prior to your appointment time.\nSee you then!
SUMMARY: 60 min massage session – Home City
END:VEVENT
END:VCALENDAR
We want to parse this file using Python code. We want to get the essential information out of this event such as: summary, description, start and end times, date, location, and if there’s any recurrence. A handy way to do so is to use the icalendar library.from icalendar import Calendar
If we want to (which I do) also reformat the date and time into more conventional formats, we will need datetime module as well.from datetime import datetime
Then we open the file and starting extracting the values from each tag from the file. For example, DTSTART precedes the time of start of appointment in a specific format. DTEND is the end time of the appointment, and so on. All content is wrapped in BEGIN and END tags. So, using the Python library, we can easily extract the values associated with each of those tags. Additionally, the times almost seem cryptic at first sight but we can display it much easier for us by some formatting in the code using strftime() function.
In the following example code, I have a handy function called parse_ical(file) that does all the parsing and displaying work (typically, I’d separate the parsing and displaying but keeping it simple here to get the concept after which you can refactor the code as you wish). The main body of the code calls this function passing it the name of an ICS/iCalender file. The output is displayed in Python shell.
Then the complete code would be:
from icalendar import Calendar
from datetime import datetime
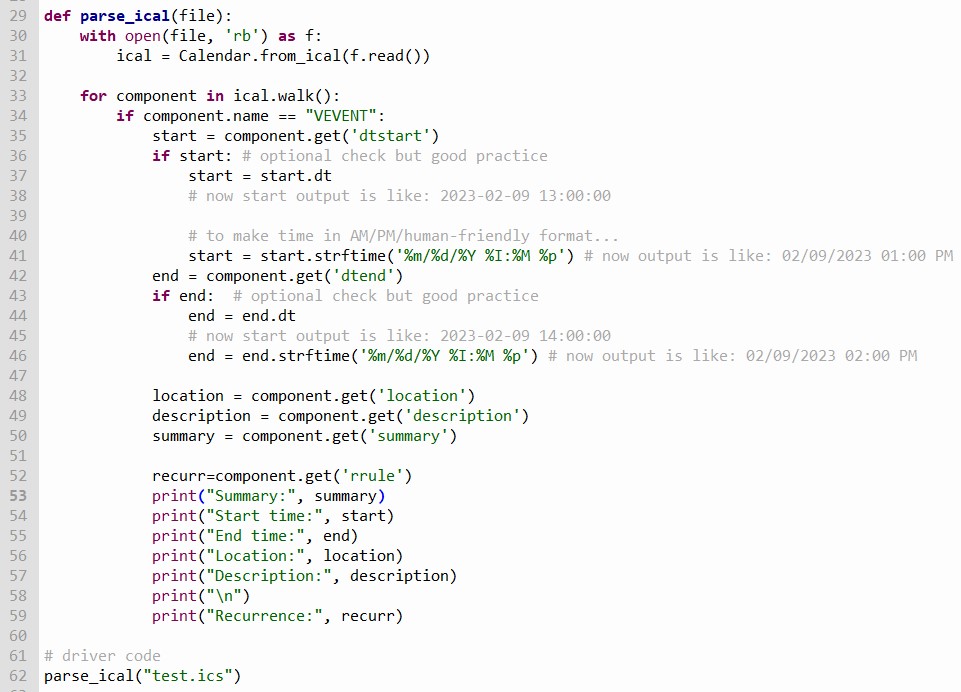
You can see above with help of my comments where we’re reformatting the date to make it user-friendly. The output of this code, when executed, is as follows:
Summary: 60 min massage session - Home City
Start time: 02/09/2023 01:00 PM
End time: 02/09/2023 02:00 PM
Location: 15111 Main Street,Home City, WA 99999
Description: 60 min massage session with Jen at 1:00 pm (555-555-5555).
Please plan to arrive 10-15 minutes prior to your appointment time.
See you then!
Recurrence: None
In the next article, I’ll share code for creating such an event from scratch (in code) and saving it into the most universal format iCalendar/ICS file.
▛Interested in creating programmable, cool electronic gadgets? Give my newest book on Arduino a try: Hello Arduino! 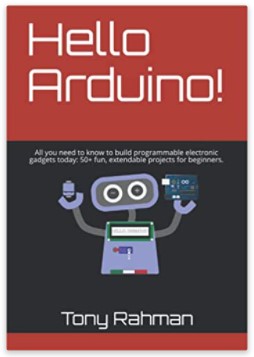
▟