In this post, I share how l implementedd the classic Bingo game (American version) in Python. This version is one player versus CPU. See the rules below if you’re not familiar with Bingo (especially American version). More details about the program and the link to the full source code below along with ability to play BINGO (run my code) right here on this site.
Rules:
A BINGO (American version) card is a 5×5 grid where each column has 5 rows and column B will have the random digits 1 to 15, column I will have digits 16-30, N will have 31-45 , G will have 46-60, and column O will have 61-75; and no column will have the same number repeated, and the center cell (cell N3) will be marked ‘X’ or ‘Free’ or ★ (‘X’ in this program).
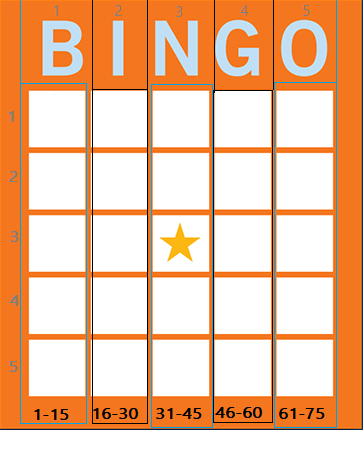
Numbers distribution on cards:
The numbers are from 1 to 75 distributed according to the columns ranges stated above. No numbers are repeated.
Cards:
Each player gets a Bingo card with random numbers (see distribution details below) spread across the 5×5 grid except the free cell (center). A player gets a card with the random numbers per rule each turn until the game is over.
Draws:
A bingo ball is randomly drawn/chosen from a total of 75 balls (1-75) each turn. If that number appears on the player’s card, that number is crossed off (by marking it with ‘X’ in this program). Once a ball is drawn from the pool of 75 balls, that number is ‘used up’ for the duration of that game and cannot be reused in the same game unti the game is quit or over.
Game over conditions:
Game ends with a win (Bingo is hit), or all 75 balls drawn. A Bingo event occurs when 5 drawn numbers (balls) are matched on the card in a row, or column, or diagonally, it’s a Bingo (win). A new game can be started by re-running the program. Each new game creates a fresh card with randomly picked numbers (as per rules above). And each ball is drawn by the CPU per turn. The program takes care of checking if the drawn ball number matches any number of the player’s card (and takes care of marking it with ‘X’ in the exact position; and if there’s no match, the program will display text saying there was no match in the console).
Win Conditions / Match Patterns:
The match patterns supported in the program are: horizontal, vertical, diagonal (top left->bottom right), diagonal (top right->bottom left). In some variations, there are other patterns…implement your version as needed from the provided source code below.
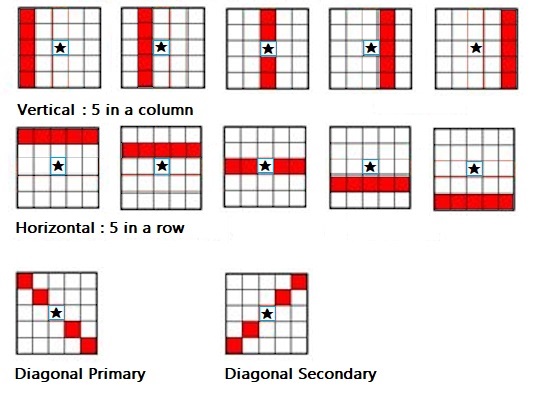
Game Play and UI:
The code is shown below. Click the > button in the box below to run the code in real-time!
To download and to run it locally on your machine, just run the python program in your IDE or in a Python runtime environment or as a EXE if converted to EXE. The program takes care of generating a card for the player, drawing each ball from the pool, checking if there is a match or not, checking which number to cross off if a match is found, handling the free cell, checking if Bingo is hit and informing the player of such, checking if all 75 balls are used up and informing the player of such, and finally the program knows when to leverage the Free cell at center to optimize a winning chance. So, the only interaction player has is to just press ENTER/RETURN key on keyboard to tell the CPU to draw the next number (ball) during the game. That’s it. Very easy to play as Bingo is really a game of luck.
When a Bingo is hit (win condition), the application will inform the player of the win along with the pattern in which the player had a bingo. e.g. *** BINGO! You win! *** Pattern: Horizontal line.
A sample session of a game where a player plays 2 turns
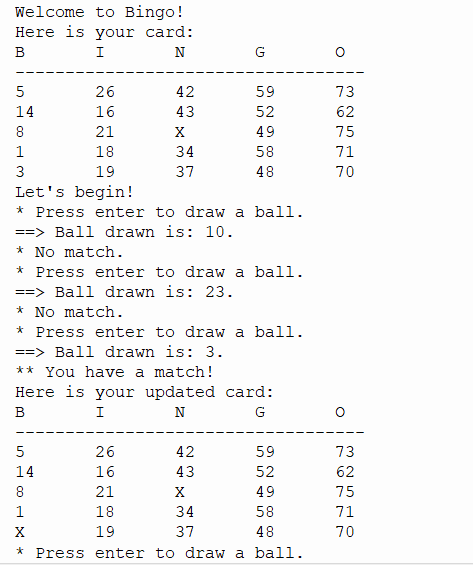
A sample session of a game where a player gets Bingo very quickly! (result not typical)
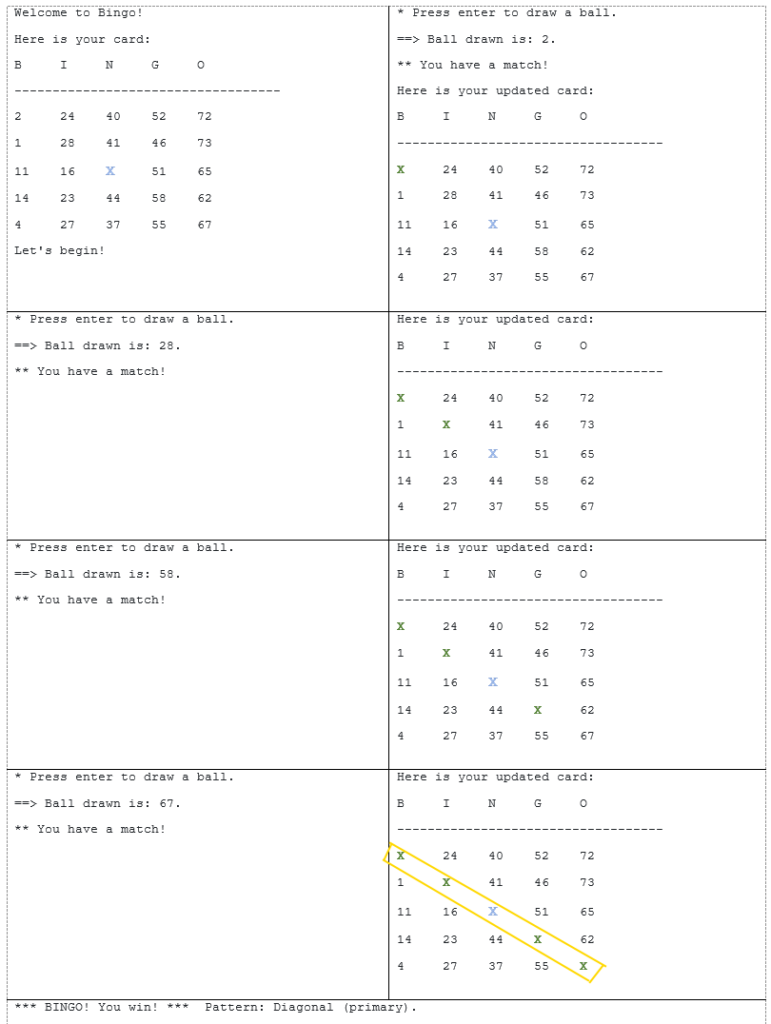
The match is a diagonal primary pattern as shown highlighted in the yellow band for explanation here. The center is a free cell, so player only had to match 4 numbers in that pattern to get a Bingo. Each turn is shown side-by-side above.
The Program:
To keep focus only on the logic and core operations of the application, no fancy graphics are used. Just plain text and console ouput. The same code can be reused with added graphics functionality for fancier UI if desired (the core code of the application can remain the same). I implemented a few helper functions to keep the code very modular and scalable.
generate_number(): Generates one number at a time for the card. Called in a loop to populate the entire card.
generate_card(): Generates all 25 numbers in a 5×5 card for all columns per call as per the columns’ ranges rules.
print_card(): Prints on console the generate card in a grid with column headers.
draw_ball(): Draws a number (aka ‘Bingo ball’) from a pool of available numbers much like a lottery ball.
check_match(): Checks if a drawn number matches any cell on player’s card.
check_bingo(): Check if player has a BINGO!
play_bingo(): Generates and displays the card per game, interacts with user, draws each number per turn, checks for match and Bingo all wrapped in a pretty while loop. This is the primary controller.
The entire source code is for anyone to view/download/run/edit on my github repo : https://github.com/flyingsalmon/bingov101
Happy learning and coding!
▛Interested in creating programmable, cool electronic gadgets? Give my newest book on Arduino a try: Hello Arduino! 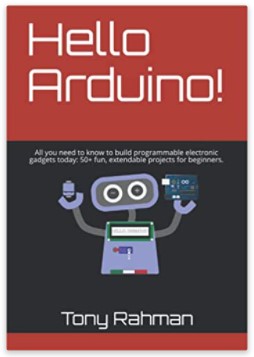
▟